Objective
The objective of this script is to detect and block brute force attacks targeting the SSH service by parsing the SSH log file (auth.log) and identifying IP addresses with multiple failed login attempts.
Introduction
Inspired by my final project, this script was developed as a proactive measure to enhance security against brute force attacks on the SSH service. By analyzing the SSH log file, I identified suspicious patterns of multiple failed login attempts originating from the same IP address. The script then utilizes UFW (Uncomplicated Firewall) to block these IP addresses, preventing further unauthorized access attempts.
Tools & Technologies Used
Bash, Linux, SSH, Network Logging, Brute Force Attacks, UFW, Iptables
Implementation
The script begins by specifying the log file to be analyzed (auth.log). It uses awk to search for lines in the log file that match three defined requirements, specifically lines containing: the same exact timestamp, "Failed password," and an IP address. The matched lines are then processed further to extract the IP addresses.
Next, awk is used again to count the occurrences of each IP address. The script filters the IP addresses based on their count, considering only those with 10 or more occurrences. These filtered IP addresses are then blocked using UFW, with the script inserting deny rules for each IP address at the top of the firewall rules.
#!/bin/bash
log_file="auth.log"
# Use awk to search for lines matching the specified requirements in the log file
matched_lines=$(awk '/[0-9]{2}:[0-9]{2}:[0-9]{2}.*Failed password.*[0-9]+\.[0-9]+\.[0-9]+\.[0-9]+/ {match($0, /[0-9]+\.[0-9]+\.[0-9]+\.[0-9]+/); print substr($0, RSTART, RLENGTH)}' "$log_file")
# Use awk to count the occurrences of each IP address
ip_counts=$(echo "$matched_lines" | awk '{ count[$0]++ } END { for (ip in count) print ip, count[ip] }')
# Filter the IP addresses based on count (10 or more occurrences)
filtered_ip_addresses=$(echo "$ip_counts" | awk '$2 >= 10 { print $1 }')
# Block the filtered IP addresses using UFW
echo "Blocking the following IP addresses with UFW:"
while read -r ip_address; do
sudo ufw insert 1 deny from "$ip_address"
echo "$ip_address"
done <<< "$filtered_ip_addresses"
Challenges & Lessons Learned
One challenge encountered during the development of this script was distinguishing between brute force attacks and legitimate login failures. To address this, I established a criteria of 10 or more failed login attempts occurring within the same second, which I deemed as highly unlikely to be the result of normal human behavior.
Through testing and experimentation, I verified that the script successfully identified and blocked IP addresses associated with brute force attacks on the SSH service.
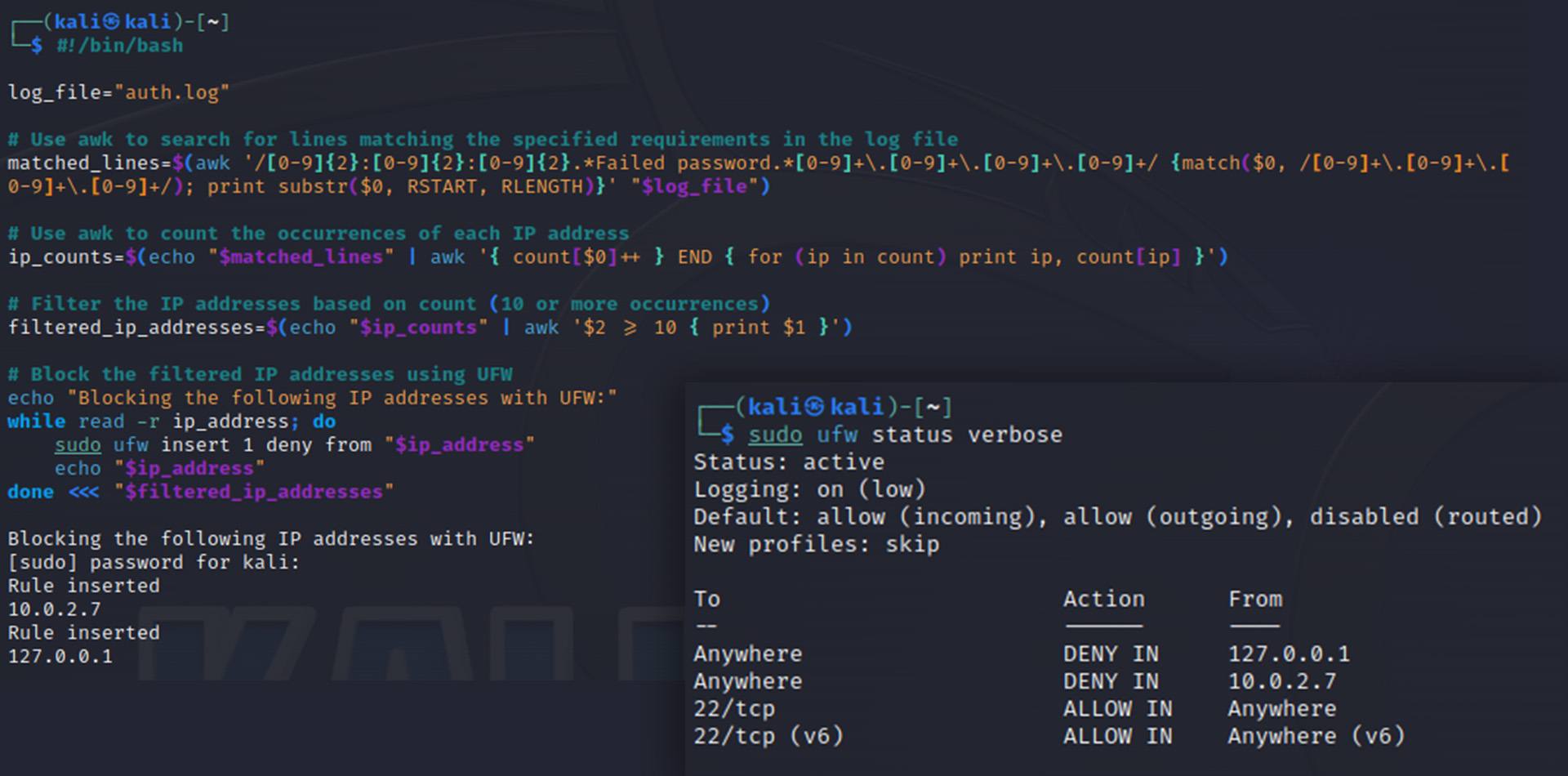
Conclusion
In conclusion, this script serves as an effective tool to detect and mitigate brute force attacks targeting the SSH service. By parsing the SSH log file and employing UFW to block malicious IP addresses, it enhances the security posture of the system and helps protect against unauthorized access attempts.
It is important to note that this script specifically targets SSH brute force attacks. To address other types of attacks or services utilizing different logs, additional scripts may be required to cover those scenarios.